Seat だけで作成したシンプルな車です。こちらはGUIを使用した方法になります。GUIを使用しない方法はこちらをご覧ください。
- Workspace に Seat を1つ追加します。
- Seat の中に RemoteEvent を追加します。
- StarterGui の中に、ScreenGui を追加します。(後でSeatの中に移動)
- その中に Frame を、さらにその中に TextButton を4つ追加します。
- Position と Size を調整して以下のように配置してください。
- 完成したら、ScreenGui を Seat の中に移動します。(座った時にGUIを表示するため)
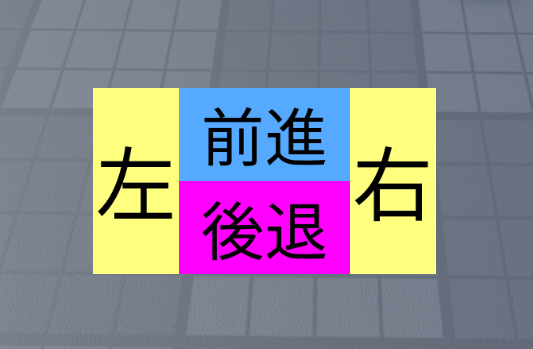
- Seat の中に Script を追加し、以下のプログラムを入力します。
local Players = game:GetService("Players")
local seat = script.Parent
local remoteEvent = seat.RemoteEvent
local currentPlayer = nil
local speed = 0
local function setupSeat()
local attachment = Instance.new("Attachment")
attachment.Parent = seat
local angularVelocity = Instance.new("AngularVelocity")
angularVelocity.Attachment0 = attachment
angularVelocity.MaxTorque = 100000
angularVelocity.Parent = seat
local linearVelocity = Instance.new("LinearVelocity")
linearVelocity.Attachment0 = attachment
linearVelocity.MaxForce = 10000
linearVelocity.Parent = seat
end
local function clearSeat()
speed = 0
seat.Attachment:Destroy()
seat.AngularVelocity:Destroy()
seat.LinearVelocity:Destroy()
end
local function setVelocity()
seat.LinearVelocity.VectorVelocity = Vector3.new(speed,speed,speed) * seat.CFrame.LookVector
end
remoteEvent.OnServerEvent:Connect(function(player,sp,turn)
if sp ~= 0 then
speed += sp
end
if turn ~= 0 then
seat.AngularVelocity.AngularVelocity = Vector3.new(0,turn,0)
wait()
seat.AngularVelocity.AngularVelocity = Vector3.new(0,0,0)
end
setVelocity()
end)
-- Seat に着席、離席したときに呼ばれる
local function onOccupantChanged()
local humanoid = seat.Occupant
if humanoid then
local character = humanoid.Parent
local player = Players:GetPlayerFromCharacter(character)
if player then
setupSeat()
local gui = seat.ScreenGui:Clone()
gui.Parent = player.PlayerGui
currentPlayer = player
return
end
end
if currentPlayer then
local gui = currentPlayer.PlayerGui:FindFirstChild("ScreenGui")
if gui then
gui:Destroy()
end
clearSeat()
currentPlayer = nil
end
end
seat:GetPropertyChangedSignal("Occupant"):Connect(onOccupantChanged)
- ScreenGui の Frame の中に LocalScript を追加し、以下のプログラムを入力します。
local seat = game.Workspace:WaitForChild("Seat")
local remoteEvent = seat:WaitForChild("RemoteEvent")
local frame = script.Parent
frame.SpeedUp.MouseButton1Click:Connect(function()
remoteEvent:FireServer(1,0)
end)
frame.SpeedDown.MouseButton1Click:Connect(function()
remoteEvent:FireServer(-1,0)
end)
frame.LeftTurn.MouseButton1Click:Connect(function()
remoteEvent:FireServer(0,1)
end)
frame.RightTurn.MouseButton1Click:Connect(function()
remoteEvent:FireServer(0,-1)
end)